Kernel Build, Module/Driver Programming & Kernel Headers – Debian Linux
Last Updated on July 13, 2023 by Hammad Rauf
I wanted to obtain the Source Code for Linux Kernel for my Debian installation, and build it just for the sake of curiosity. I will document here the steps I used for Kernel sources download, and build. I also explored writing a simple Module and loading/unloading it in the installed and running Kernel. You may wish to read my older post about how to install the Linux Mint Debian Edition (LMDE) with the latest Kernel binary image, before continuing.
Contents
Debian Kernel Build
Sub-Contents
- What is Kernel?
- What is Make?
- Download Sources for Kernel and Configure for Build
- Start the Build
What is Kernel?
Kernel is the core system software in a Linux Operating System. It is responsible for managing system resources, such as memory and CPU allocation, and providing a bridge between application software and hardware. The kernel also provides services to user-space programs, such as system calls, and acts as an abstraction layer between the computer’s hardware and the software that runs on it. Linux Kernel is open source software, meaning that its Source Code is available publicly and can be easily downloaded, modified and compiled by anyone. It is mostly written in C/C++ programming languages.
What is Make?
The “make” tool is a program that is commonly used by C/C++ programmers to automate the process of building and compiling software. It uses a “makefile” (a simple text file containing a set of rules) to determine how to build the software. The “makefile” specifies how source code files are to be transformed into executable programs, and describes the dependencies between the source code files. When you run the “make” command, it reads the “makefile” and automatically builds the software in the correct order, using the appropriate commands to compile and link the source code files. This can save a lot of time and effort, as the “makefile” can be set up to automatically handle tasks such as dependency checking, parallel builds and it can also handle cross-compilation.
Download Sources for Kernel and Configure for Build
You will need to enable the sources repositories for LMDE Linux, so that it can locate the source code files for your version of the Kernel.
To enable the LMDE Installation to search for source code do the following:
OS Key (Or Start Menu) –> Software Sources –> Official Repositories –> Enable (Source Code Repositories, Debug Symbols). {Screen Shot}
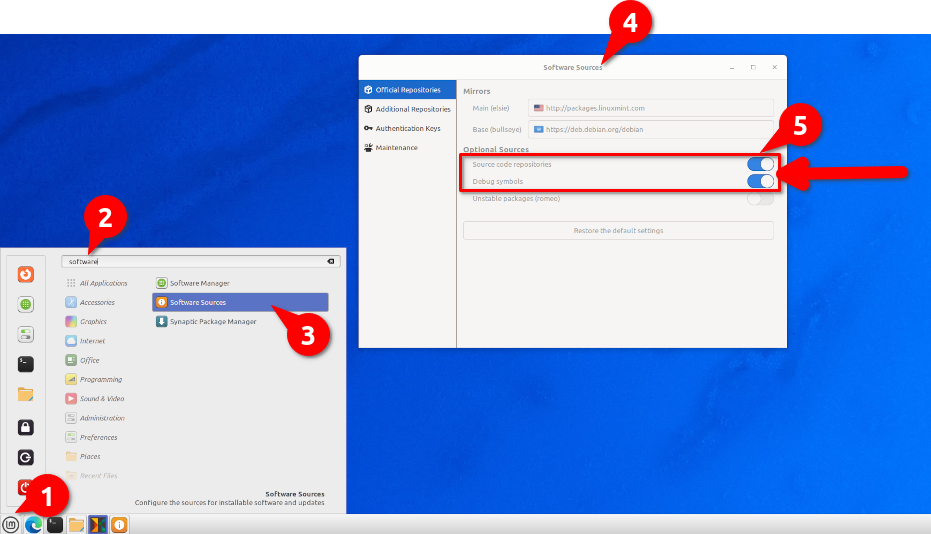
Use the following sequence of commands to download Debian Linux source code
cd ~/Downloads sudo apt-get install build-essential fakeroot rsync git sudo apt-get build-dep linux apt-get source linux cd linux-version # OR cd linux-6.0.12
Start the Build
Use the following commands to start the Build process. Once started it can take several hours to compile the Kernel.
cd ~/Downloads/linux-version ## OR cd ~/Downloads/linux-6.0.12 debian/rules orig debian/rules debian/control ## Make Changes in Loaded Modules, etc. OR Just examine only.... make menuconfig # OR # make all # make nconfig # make xconfig, etc. ## NOW COMPILE fakeroot debian/rules binary
Writing a Simple Module
Sub-Contents
- What is a Module?
- Sample Hello Message Module Code
- Compile Module
- Load and Unload Module
What is a Module?
In Linux kernel, a module is a piece of code that can be loaded and unloaded into the kernel at runtime. This allows the kernel to be extended and modified without having to be completely rebuilt. Modules are typically used to add support for new hardware devices or to provide new kernel functionality.
Each module has its own set of functions and data structures that can be accessed by other parts of the kernel. When a module is loaded, it is given a unique identifier and added to a list of currently loaded modules. Once a module is loaded, it can be used by other parts of the kernel and can also register itself to be notified of certain events or to receive certain data.
Modules can be loaded and unloaded using the “insmod” and “rmmod” commands, respectively. The kernel also provide a feature called “Kernel Probes” which allows for dynamic instrumentation of the kernel, and can be used for tracing, debugging and performance analysis.
The module also have their own versioning, dependencies and license information, which can be queried using the “modinfo” command.
Sample Hello Message Module Source Code
The following C source code defines a simple “Hello World!” type basic module, that is actually doing nothing more than demonstrating how modules can be compiled and loaded in Linux kernel.
// Save as filename: hello.c #include <linux/init.h> #include <linux/module.h> // Module metadata MODULE_AUTHOR("Hammad Rauf"); MODULE_DESCRIPTION("Hello world Module."); MODULE_LICENSE("GPL"); static int hello_init(void) { printk(KERN_INFO "Hello, world! The Module has been loaded.\n"); return 0; } static void hello_exit(void) { printk(KERN_INFO "Goodbye, cruel world. Module unloading.\n"); } module_init(hello_init); module_exit(hello_exit);
The following “Makefile” will take care of compiling this module properly. You will need to download and install Linux Kernel Headers (See the next section in this post), before issuing the make command.
obj-m += hello.o PWD=$(shell pwd) VER=$(shell uname -r) KERNEL_BUILD=/lib/modules/$(VER)/build all: make -C /lib/modules/$(shell uname -r)/build M=$(PWD) modules clean: make -C /lib/modules/$(shell uname -r)/build M=$(PWD) clean
Make sure that the above 2 files are saved in one folder.
Compile A Module
To compile the sample Module change into the folder where you copied the files from previous sub-section. Then type the following command:
make # OR make all
In case of errors you may want to delete all generated files by issuing the command:
make clean
Load and Unload Module
In Debian Linux, you can load a kernel module using the “modprobe” command. The “modprobe” command is used to add or remove a module from the Linux kernel.
To load a module, you can use the following syntax:
sudo modprobe MODULE_NAME
For example, to load the “hello” module, you would use the following command:
sudo modprobe hello
Alternatively, you can use the “insmod” command, which is the older command to insert modules into the kernel, but it is less recommended than “modprobe” because it does not handle dependencies and other configurations.
sudo insmod /path/to/MODULE_NAME.ko
Once a module is loaded, you can check if it is loaded by using the “lsmod” command, which displays the status of modules in the Linux kernel.
lsmod | grep MODULE_NAME
You can also use the “modinfo” command to get information about a module, such as the version, author, and description.
modinfo MODULE_NAME
Please note that you need to have root privilege or use “sudo” command to execute the above commands.
Install Kernel Headers
Sub-Contents
- What are Kernel Headers and Why are they needed?
- Installing the Kernel Headers
What are Kernel Headers and Why are they needed?
Linux kernel headers are a collection of header files that provide the interface between the Linux kernel and user-space programs. These header files define the data structures, macros, and function prototypes that are used by the kernel and by the system libraries that provide system calls to user-space programs. Header files are a way for C/C++ programmers to package source code data definitions and source code instructions separately from each other. One of the uses of Header files, by using Header files source code instructions can be changed easily by programmers, while the Headers (source code data definitions) remain unchanged, and in turn may not break other dependent parts of the Operating System.
The headers are typically located in the “/usr/src/linux-headers-version-architecture” directory, where version is the version of the kernel and architecture is the architecture of the system (e.g. x86_64, amd64, arm64, etc.).
These headers are used by developers to write kernel modules, device drivers, and other software that needs to interact with the kernel. They are also used by system libraries and user-space programs that need to make system calls to the kernel. When compiling custom Linux Kernel Modules, you will be needed Kernel Headers on your local Linux installation.
The headers are also used when building the kernel module, and are required to build some other software such as VirtualBox, VMware, and nvidia graphics driver that need to interact with kernel.
Installing the Kernel Headers
You can install the headers package for your specific version of the kernel by using package manager of your Linux distribution, for example in Ubuntu or Debian you can use the command
sudo apt-get install linux-headers-$(uname -r)
Further Reading
- https://andromedabay.ddns.net/install-debian-linux-on-x86-amd64-computer/, Install LMDE on a x86/AMD64 computer with latest Kernel binary, Date Accessed: January 1, 2023
- https://www.debian.org/doc/manuals/debian-kernel-handbook/ch-common-tasks.html, Debian Kernel Handbook – Common Tasks, Date Accessed: January 16, 2023
- https://medium.com/dvt-engineering/how-to-write-your-first-linux-kernel-module-cf284408beeb, How to write your first linux kernel module, Date Accessed: January 16, 2023
- https://stackoverflow.com/questions/3140478/fatal-module-not-found-error-using-modprobe StackOverflow Question Example Module, Date Accessed: January 16, 2023